
Led Spot Ampul Fiyatları-Led Spot Ampul Toptan Fiyat Listesi;Led spot ampul dayanıklı led spot ampul profesyonel led spot ampul kaliteli led spot ampul imalatçıları led spot ampul fiyatı led spot ampul tasarruflu
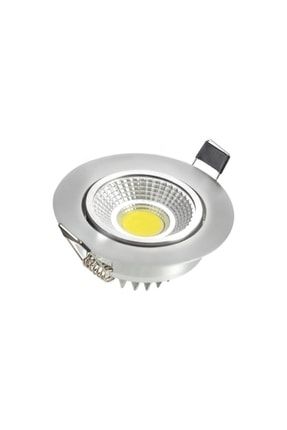
özmr lighting Garra Cob Krom Kasa Beyaz Işık Trafolu 5 Watt Led Spot Armatür (1 ADET) Fiyatı, Yorumları - Trendyol
